emRun
The C runtime library for embedded applications
emRun is a complete C runtime library specifically designed and optimized for GCC/LLVM-based toolchains and embedded systems.
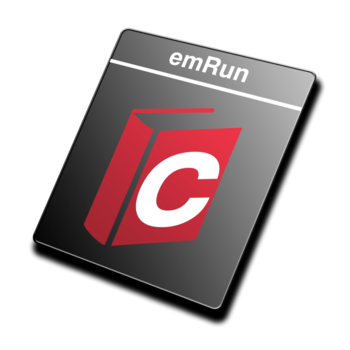
Overview
Most toolchains using GCC or LLVM also use either newlib, newlib-nano or glibc. Unfortunately, these libraries have significant disadvantages over professional runtime libraries for embedded systems. This is where emRun comes in. It can be used with GCC, LLVM, and other toolchains to provide a runtime library designed and engineered for embedded systems. Written from the ground up for embedded devices, emRun enables a high degree of optimization with low requirements.
emRun is a complete C runtime library for use with any toolchain. It converts any GCC/LLVM-based toolchain into a professional development choice. emRun is used in SEGGER's Embedded Studio IDE and has proven its value for years.
- Highly optimized functions that, when used, avoid the inclusion of additional support functions (e.g. printf) that are aren’t needed
- Formatted input and output functions are customizable from basic I/O to fully featured I/O, enabling reduction of final code footprint
- Full support for localization, UTF-8, code pages, and locale codecs is only linked if used
In many cases, the ROM-savings of emRun enable the use of a smaller microcontroller with less on-chip memory. This can result in significant cost savings, especially for devices built in large quantities for the mass market.
Being a simple replacement for libraries, such as newlib or newlib-nano, emRun shrinks and accelerates embedded applications. Whether the main concern with newlib is flash size, performance, or the attribution clause of the viral licensing, the SEGGER Runtime Library emRun is the solution.
Key features
- High performance, with time-critical routines written in assembly language
- Significant code size reduction
- Configurable for high speed or small size
- Includes SEGGER's optimized floating-point library emFloat
- Designed for use with various toolchains
- EABI compatible functions
- Minimum RAM usage
- No heap requirements
- No viral licensing, no attribution clause
Licensing
emRun can be licensed in source code to individual companies as well as be included as libraries by providers of toolchains.
- Source code makes it possible to step through library functions
- Many functions can be tuned with different implementations for size-optimized code and speed-optimized code
emRun is also available in generic "C" code to run on any system. Additionally assembly optimized variants are available for:
- Arm (Cortex-M and Cortex-A 32-bit)
- RISC-V (RV32M)
Ports for other CPUs can be made available on request. Please contact us for more information.
Silicon vendor buyout option
SEGGER offers vendors the option to license emRun for redistribution to their own customers under their own terms. Please contact us to complete your offerings with a proven commercial solution.
Who offers emRun performance?
The reference platforms for emRun are Embedded Studio for Arm and Embedded Studio for RISC-V. Embedded Studio can be used to easily evaluate performance, free of charge. In addition, a number of vendors have adopted the SEGGER runtime library. It has been integrated into the IDEs of the following (as well as by other larger corporations):
Performance tuning — speed and size
The performance of a runtime library can have a huge impact on the speed of an application. Despite trying to avoid library functions (sprintf(), memcpy(), memcmp() etc) the compiler will make you call library functions, such as for division and multiplication, that you do not see, especially when dealing with floating point or 64-bit operations, Here the choice of the right runtime library makes all the difference.
emRun uses enhanced, highly advanced low-level implementations for such operations, which can be fine-tuned for speed or size. With the assembly-optimized variants, performance can be even more optimized by using the target platform to its full potential.
Discover this and more in our blog articles:
- Every byte counts — Smallest “Hello world”
- Every byte counts — The 100-Byte Blinky challenge
- Every byte counts — Floating point in less than 1 KB
- Algorithms for division — Part 1
- Algorithms for division — Part 2— Classics
- Algorithms for division — Part 3 — Using multiplication
- Algorithms for division — Part 4 — Using Newton’s method
- Saving power in embedded systems — Reducing idle CPU speed
Performance
RISC-V (32-Bit)
Runtime [Cycles] | |||
---|---|---|---|
Test Project [1] | emRun [2] | gcc / newlib [3] | |
Floating-Point Arithmetic | 1097.4 | 1907.0 | |
Floating-Point Math | 714.03 | 1814.36 | |
Integer Arithmetic | 251.2 | 1041.77 | |
String and Memory Functions | 17141 | 20733 |
RISC-V (64-Bit)
Runtime [Cycles] | |||
---|---|---|---|
Test Project [1] | emRun [4] | gcc / newlib [5] | |
Floating-Point Arithmetic | 826.8 | 1684.4 | |
Floating-Point Math | 734.71 | 1712.70 | |
Integer Arithmetic | 19.0 | 1560.6 | |
String and Memory Functions | 10430 | 17633 |
Arm (32-Bit)
Runtime [Cycles] | |||
---|---|---|---|
Test Project [1] | emRun [6] | gcc / newlib [7] | |
Floating-Point Arithmetic | 821.0 | 2618.8 | |
Floating-Point Math | 544.32 | 1003.35 | |
Integer Arithmetic | 122.3 | 195.7 | |
String and Memory Functions | 10696 | 17332 |
[1] Source code and more information available at https://wiki.segger.com/emRun
[2] SEGGER Embedded Studio for RISC-V version 6.40, GNU Toolchain, emRun 4.22.0, RV32IMAC
[3] SEGGER Embedded Studio for RISC-V version 6.34, GNU Toolchain, libgcc 12.1.0 and newlib 4.1.0, RV32IMAC
[4] SEGGER Embedded Studio for RISC-V version 6.40, GNU Toolchain, emRun 4.22.0, RV64IMAC
[5] SEGGER Embedded Studio for RISC-V version 6.34, GNU Toolchain, libgcc 12.1.0 and newlib 4.1.0, RV64IMAC
[6] SEGGER Embedded Studio for ARM version 6.34, GNU Toolchain, emRun 4.18.0, ARMv7M
[7] SEGGER Embedded Studio for ARM version 6.34, GNU Toolchain, libgcc 9.0 and newlib 3.3.0, ARMv7M
Memory requirements
emRun offers significant savings in flash memory. This is partly due to some functions being hand-coded in assembly language, but primarily due to a structure that minimizes internal library dependencies, for example the file I/O not being linked in with printf() or scanf().
emRun also uses less static RAM due to a structure designed from the ground up for embedded systems.
Benchmark code:
int main(void) {
printf("Hello World.\n");
return 0;
}
SEGGER did some benchmarking on emRun using NXP’s MCUXpresso as a representative IDE that might benefit from using emRun. The benchmark consists of a simple "printf()" with output to a debug terminal. We compared the results for emRun with the results for three other C libraries (Newlib, Newlib-nano, and Redlib). In each case, printf() was configured for the smallest possible size (size-optimized libraries, no floating point...). We then also tested with our own IDE, Embedded Studio, with the same code, using emRun, in one case using the GNU Linker, in another case using the SEGGER Linker.
Test Environment | Test project: Simple "main" project with "printf()" | |||
---|---|---|---|---|
ROM Usage | RAM Usage | |||
MCUXpresso1, Newlib | 31888 bytes | 2864 bytes | ||
MCUXpresso, Redlib | 9940 bytes | 728 bytes | ||
MCUXpresso, Newlib-nano | 9552 bytes | 416 bytes | ||
MCUXpresso, SEGGER RunTime Library | 4392 bytes | 184 bytes | ||
Embedded Studio2, SEGGER emRun, GNU Linker | 2502 bytes | 0 bytes | ||
Embedded Studio, SEGGER emRun, SEGGER Linker | 1868 bytes | 0 bytes | ||
Embedded Studio, SEGGER emRun, SEGGER Linker, Host Formatting | 818 bytes | 8 bytes |
1: MCUXpresso IDE v3.1.0-2200 and SDK 2.5.0 for the Freedom K66F board
2: Embedded Studio 4.16 with the Kinetis K60 CPU Support Package v1.02
Memory usage
RISC-V (32-Bit)
ROM Usage | |||
---|---|---|---|
Test Project [1] | emRun [2] | gcc / newlib [3] | |
Floating-Point Arithmetic | 12795 | 21077 | |
Floating-Point Math | 21496 | 69096 | |
Integer Arithmetic | 2744 | 6068 | |
String and Memory Functions | 5762 | 6606 |
RISC-V (64-Bit)
ROM Usage | |||
---|---|---|---|
Test Project [1] | emRun [4] | gcc / newlib [5] | |
Floating-Point Arithmetic | 15137 | 17387 | |
Floating-Point Math | 19830 | 60922 | |
Integer Arithmetic | 1786 | 1940 | |
String and Memory Functions | 5878 | 6844 |
[1] Source code and more information available at https://wiki.segger.com/emRun
[2] SEGGER Embedded Studio for RISC-V version 6.34, GNU Toolchain, emRun 4.18.0, RV32IMAC
[3] SEGGER Embedded Studio for RISC-V version 6.34, GNU Toolchain, libgcc 12.1.0 and newlib 4.1.0, RV32IMAC
[4] SEGGER Embedded Studio for RISC-V version 6.34, GNU Toolchain, emRun 4.18.0, RV64IMAC
[5] SEGGER Embedded Studio for RISC-V version 6.34, GNU Toolchain, libgcc 12.1.0 and newlib 4.1.0, RV64IMAC
Implementation & configuration
emRun can be used with any GCC-based IDE/toolchain by replacing the runtime library delivered with that toolchain (usually newlib, newlib-nano, or some other variant) with emRun.
Examples include:
- AC6 System Workbench for STM32 (SW4STM32)
- Atmel Studio 7
- Atollic TrueStudio for STM32
- NXP MCUXpresso
- Renesas e2 studio (for Synergy or RZ targets)
- SiLabs Simplicity Studio
In SEGGER Embedded Studio and Rowley CrossWorks, emRun is already included as the default C library.
Please see the user guide for details.
Library verification
To verify the functionality of emRun, we created and used our verification test suite. It checks the entire functionality of all library functions, including the entire floating point library with all corner cases.
More than 450 different tests with millions of test cases are executed. Below an exert for just a single test for sys_float64_add(), adding 64-bit doubles:
If you are interested in replaying our results, or want to verify the library you currently use, or plan to develop your own runtime library, the SEGGER Runtime Library Verification Test Suite is available.
emFloat — Optimized floating-point library
A key component of emRun is emFloat, a highly optimized, IEEE 754 compliant, floating-point library designed from the ground up for embedded systems. Very fast and very small, it delivers FPU-like performance in pure software. Even where an FPU is available, emFloat boosts the FPU’s performance for complex mathematical functions.